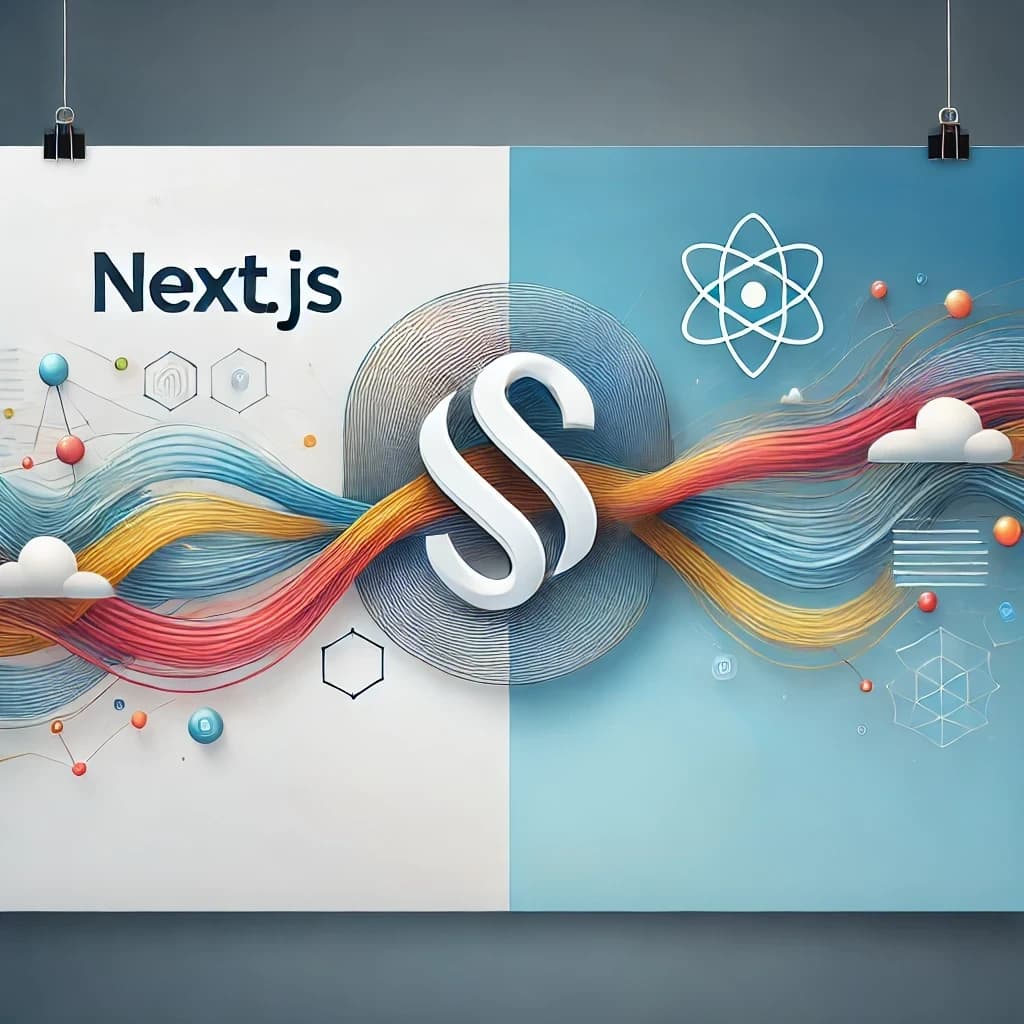
How to Integrate Contentful with Next.js: A Complete Guide
In modern web development, content management systems (CMS) are essential for delivering dynamic, data-driven websites. Contentful is a powerful headless CMS that allows developers to manage content separately from their code. Combined with a React-based framework like Next.js, Contentful enables the creation of fast, scalable, and content-rich web applications.
In this tutorial, we'll walk through how to integrate Contentful with a Next.js application to create a seamless experience where content is dynamically fetched and rendered on your website.
Table of Contents
- What is Contentful?
- Setting Up Contentful
- Setting Up the Next.js Project
- Installing Required Packages
- Fetching Content from Contentful
- Rendering Content in Next.js Pages
- Handling Dynamic Routes
- Deploying Your Application
- Conclusion
What is Contentful?
Contentful is a cloud-based headless CMS that allows you to create, manage, and deliver content across multiple platforms. Unlike traditional CMSs (e.g., WordPress), which manage both the content and presentation, Contentful only manages the content. This makes it a perfect fit for modern front-end frameworks like Next.js, which handle the rendering of content on the client side.
In Contentful, content is structured in "content types" and can include rich text, images, videos, and more. You can fetch this content via Contentful's RESTful APIs or GraphQL endpoints, and use it to populate your front-end application.
Setting Up Contentful
Before integrating Contentful with your Next.js app, you need to set up your Contentful account and create your first space (a container for your content).
Step 1: Create a Contentful Account
- Visit Contentful and sign up for a free account.
- Once logged in, create a new Space. A space represents a single project or website, and it contains all your content.
Step 2: Create Content Models
In Contentful, you organize content using "content types." Each content type represents a type of content (e.g., Blog Post, Author, etc.). For this tutorial, let's assume we're building a blog site.
- Go to the Content Model tab and create a new content type called
Blog Post
. - Add fields like:
- Title (Text field)
- Body (Rich Text field)
- Slug (Text field, for unique URL slugs)
- Published Date (Date field)
- Save your content model.
Step 3: Add Content
Once your content type is created, you can add entries:
- Go to the Content tab and click Add Entry under the
Blog Post
content type. - Fill out the fields with the title, body, slug, and published date.
- Save the entry.
Step 4: Get API Credentials
To access the content programmatically, you need an API key.
- In the Contentful dashboard, go to Settings > API keys.
- Create a new API key. You'll get a
Space ID
and anAccess Token
. Keep these safe as you'll need them to connect your Next.js app with Contentful.
Setting Up the Next.js Project
Next.js is a React framework that provides features like server-side rendering (SSR), static site generation (SSG), and API routes. To get started, you'll need to set up a new Next.js project.
Step 1: Create a Next.js App
Run the following commands to set up a new Next.js application:
npx create-next-app contentful-nextjs
cd contentful-nextjs
Step 2: Install Dependencies
Next, install the contentful
package, which provides a JavaScript SDK to interact with the Contentful API.
npm install contentful
Installing Required Packages
At this point, we've created the Next.js app and installed the necessary dependencies. Now we need to configure Contentful in the app.
Step 1: Create Environment Variables
Create a .env.local
file in the root of your project and add your Contentful SPACE_ID
and ACCESS_TOKEN
:
CONTENTFUL_SPACE_ID=your_space_id
CONTENTFUL_ACCESS_TOKEN=your_access_token
Make sure to replace your_space_id
and your_access_token
with the actual values from your Contentful dashboard.
Step 2: Set Up the Contentful Client
Create a new file lib/contentful.js
to set up the Contentful client:
// lib/contentful.js
import { createClient } from 'contentful';
export const client = createClient({
space: process.env.CONTENTFUL_SPACE_ID,
accessToken: process.env.CONTENTFUL_ACCESS_TOKEN,
});
Fetching Content from Contentful
To fetch content from Contentful, you'll use the Contentful client to retrieve entries based on a query.
Step 1: Fetching Content for Static Generation (SSG)
Next.js offers Static Site Generation (SSG), which is ideal for fetching data at build time. You can fetch blog posts using getStaticProps()
to pre-render content.
Create a new file pages/index.js
:
// pages/index.js
import { client } from '../lib/contentful';
export async function getStaticProps() {
// Fetch all blog posts
const response = await client.getEntries({ content_type: 'blogPost' });
return {
props: {
posts: response.items,
},
};
}
export default function Home({ posts }) {
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.sys.id}>
<h2>{post.fields.title}</h2>
<p>{post.fields.body}</p>
</li>
))}
</ul>
</div>
);
}
In this example, getStaticProps()
fetches all blog posts from Contentful at build time, and the posts are rendered on the homepage.
Step 2: Fetching a Single Blog Post
To fetch a single blog post, you can use dynamic routing in Next.js. For this, you'll need getStaticPaths()
and getStaticProps()
.
Create a file pages/posts/[slug].js
:
// pages/posts/[slug].js
import { client } from '../../lib/contentful';
export async function getStaticPaths() {
const response = await client.getEntries({ content_type: 'blogPost' });
const paths = response.items.map((post) => ({
params: { slug: post.fields.slug },
}));
return {
paths,
fallback: false,
};
}
export async function getStaticProps({ params }) {
const { slug } = params;
const response = await client.getEntries({
content_type: 'blogPost',
'fields.slug': slug,
});
return {
props: {
post: response.items[0],
},
};
}
export default function Post({ post }) {
return (
<div>
<h1>{post.fields.title}</h1>
<div>{post.fields.body}</div>
</div>
);
}
In this case, getStaticPaths()
dynamically generates paths for each blog post, and getStaticProps()
fetches the individual post based on the slug
parameter.
Deploying Your Application
Once your app is working locally, you can deploy it to platforms like Vercel (which is the creator of Next.js) for easy deployment and hosting.
Step 1: Deploy to Vercel
- Go to Vercel and sign up.
- Connect your GitHub repository and deploy your Next.js app.
- Vercel will automatically handle environment variables for you. Just make sure to add your Contentful
SPACE_ID
andACCESS_TOKEN
in the Vercel dashboard.
Conclusion
Integrating Contentful with Next.js allows you to create dynamic websites that fetch and display content from a headless CMS. This combination provides a powerful and flexible solution for building modern, content-driven applications.
In this tutorial, we've walked through how to set up Contentful, integrate it into a Next.js project, and fetch both static and dynamic content. From here, you can expand your project by adding more complex content types, optimizing the performance, and deploying it to production.
Happy coding!